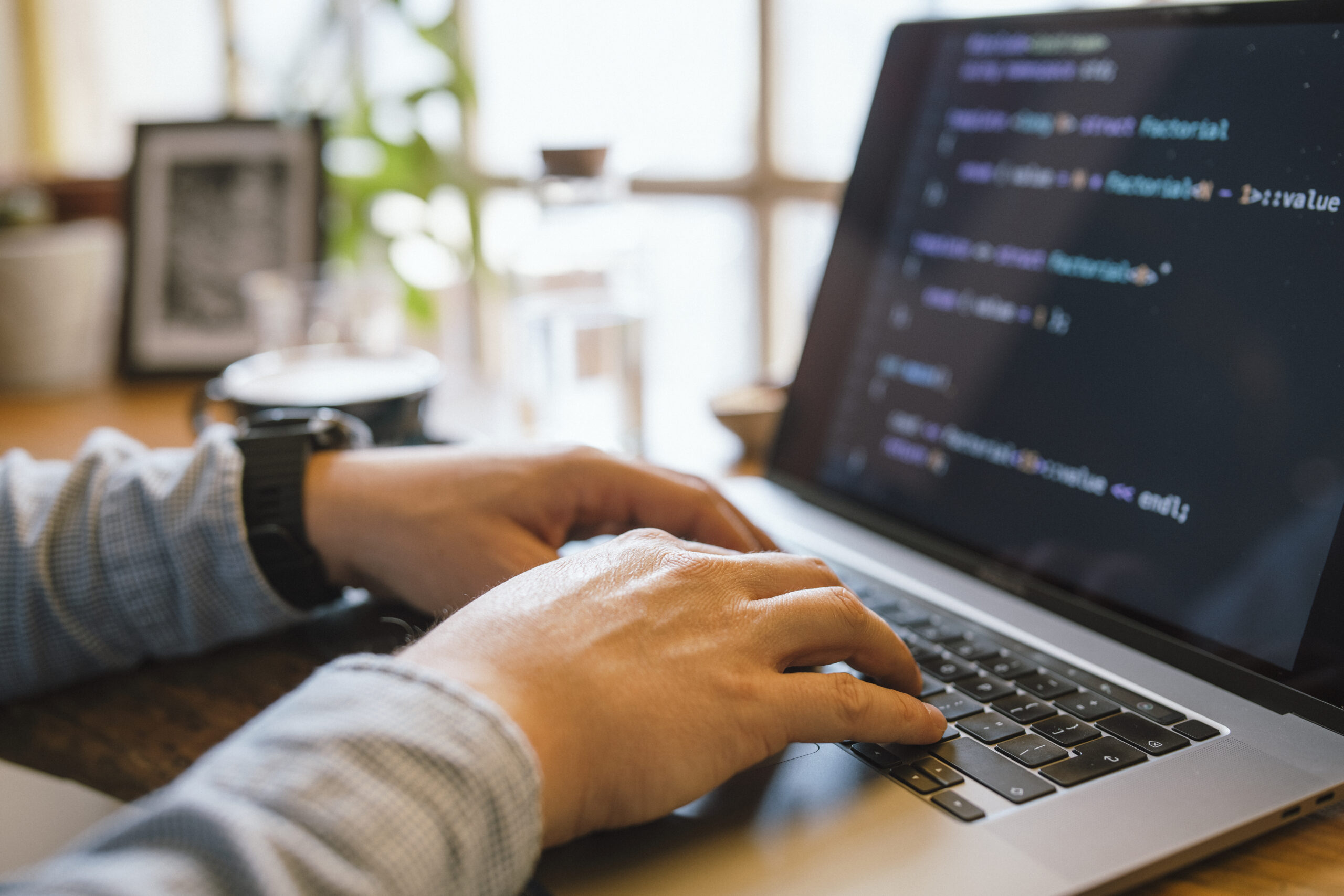
Debugging is Just about the most necessary — yet usually neglected — competencies in a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why issues go Improper, and Discovering to Believe methodically to solve difficulties proficiently. No matter if you are a starter or simply a seasoned developer, sharpening your debugging abilities can save hours of aggravation and significantly boost your productivity. Listed here are a number of methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest techniques developers can elevate their debugging skills is by mastering the tools they use every day. Though producing code is one particular Portion of advancement, realizing how you can interact with it proficiently for the duration of execution is equally important. Fashionable advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these instruments can do.
Acquire, one example is, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and also modify code on the fly. When applied properly, they Permit you to observe accurately how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude developers. They help you inspect the DOM, keep an eye on network requests, watch genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can convert annoying UI issues into workable duties.
For backend or procedure-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control in excess of running processes and memory administration. Studying these instruments may have a steeper Understanding curve but pays off when debugging general performance troubles, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be snug with Edition Regulate techniques like Git to be familiar with code record, locate the precise instant bugs had been launched, and isolate problematic variations.
In the end, mastering your equipment usually means going past default settings and shortcuts — it’s about building an intimate familiarity with your progress ecosystem to make sure that when issues arise, you’re not misplaced at midnight. The better you understand your equipment, the greater time you may shell out fixing the actual dilemma in lieu of fumbling by the method.
Reproduce the situation
One of the more significant — and infrequently forgotten — techniques in powerful debugging is reproducing the situation. Right before leaping to the code or producing guesses, developers have to have to produce a regular setting or situation where the bug reliably seems. With no reproducibility, fixing a bug gets to be a sport of prospect, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What steps brought about the issue? Which natural environment was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered ample details, seek to recreate the situation in your local natural environment. This might necessarily mean inputting the identical data, simulating related person interactions, or mimicking technique states. If The difficulty appears intermittently, take into consideration composing automatic tests that replicate the sting cases or condition transitions associated. These exams not only aid expose the problem but in addition prevent regressions in the future.
From time to time, the issue may very well be surroundings-unique — it might come about only on sure operating programs, browsers, or less than specific configurations. Making use of applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a move — it’s a state of mind. It calls for tolerance, observation, and a methodical strategy. But as soon as you can continually recreate the bug, you might be already halfway to repairing it. Which has a reproducible situation, You need to use your debugging applications more efficiently, take a look at probable fixes properly, and talk a lot more Obviously along with your crew or buyers. It turns an summary criticism right into a concrete obstacle — Which’s the place developers prosper.
Browse and Comprehend the Error Messages
Error messages are frequently the most useful clues a developer has when a thing goes Erroneous. Rather then looking at them as discouraging interruptions, builders must find out to treat error messages as immediate communications within the technique. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Quite a few developers, especially when underneath time strain, glance at the 1st line and quickly begin earning assumptions. But deeper within the mistake stack or logs may possibly lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them initial.
Crack the error down into areas. Is it a syntax mistake, a runtime exception, or a logic mistake? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging method.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the error transpired. Test similar log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These typically precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more effective equipment in the developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for common events (like thriving get started-ups), Alert for prospective problems that don’t crack the applying, Mistake for real problems, and Lethal if the program can’t continue.
Stay away from flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and slow down your process. Give attention to important situations, condition modifications, enter/output values, and demanding decision factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what problems are fulfilled, and what branches of logic are executed—all without having halting This system. They’re Specifically beneficial in output environments in which stepping through code isn’t attainable.
Furthermore, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. Which has a effectively-assumed-out logging technique, you are able to lessen the time it takes to identify concerns, attain deeper visibility into your programs, and Increase the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not merely a technical activity—it is a sort of investigation. To effectively recognize and deal with bugs, builders must method the method similar to a detective resolving a mystery. This attitude will help stop working elaborate challenges into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating evidence. Look at the indicators of the situation: mistake messages, incorrect output, or performance problems. Much like a detective surveys a criminal offense scene, acquire just as much applicable information as you can with out jumping to conclusions. Use logs, test cases, and user reports to piece together a transparent photograph of what’s occurring.
Upcoming, kind hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications recently been made into the codebase? Has this difficulty transpired ahead of below comparable circumstances? The intention will be to slim down choices and identify potential culprits.
Then, exam your theories systematically. Try and recreate the trouble inside a managed setting. Should you suspect a specific purpose or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects direct you closer to the reality.
Spend shut focus to small information. Bugs frequently disguise inside the the very least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and affected person, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the real trouble, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, solution issues methodically, and turn into more practical at uncovering hidden problems in intricate units.
Create Exams
Producing checks is among the most effective solutions to improve your debugging abilities and All round progress performance. Checks not only assist catch bugs early but in addition function a security Internet that offers you assurance when creating adjustments in your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device checks, which deal with unique capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly reducing some time expended debugging. Unit exams are Particularly useful for catching regression bugs—challenges that reappear just after Earlier getting set.
Next, combine integration assessments and finish-to-end checks into your workflow. These assistance be sure that a variety of elements of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what conditions.
Composing tests also forces you to definitely think critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust initial step. When the test fails persistently, you can give attention to correcting the bug and watch your examination move when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable procedure—supporting you capture more bugs, quicker and a lot more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to be immersed in the condition—looking at your display for hrs, seeking solution following Remedy. But Among the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your mind, decrease disappointment, and sometimes see The problem from a new viewpoint.
When you are way too near to the code for far too very long, cognitive exhaustion sets in. You may perhaps start overlooking obvious mistakes or misreading code that you just wrote just hrs previously. In this particular condition, your Mind results in being fewer economical at trouble-resolving. A brief stroll, a espresso split, and even switching to a distinct job for 10–15 minutes can refresh your focus. Many builders report acquiring the basis of a difficulty after they've taken time to disconnect, permitting their subconscious get the job done from the track record.
Breaks also aid avoid burnout, Specifically throughout lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed Power and a clearer mentality. You would possibly abruptly discover a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
If you’re caught, a great general guideline is always to established a here timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move around, extend, or do some thing unrelated to code. It may well come to feel counterintuitive, Particularly less than restricted deadlines, but it essentially results in faster and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible method. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is really a psychological puzzle, and relaxation is part of fixing it.
Master From Every Bug
Just about every bug you encounter is more than simply A short lived setback—it's a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural concern, each one can educate you anything precious if you take some time to mirror and examine what went Erroneous.
Start by asking oneself a number of critical thoughts once the bug is settled: What brought about it? Why did it go unnoticed? Could it are already caught previously with greater tactics like device testing, code critiques, or logging? The answers usually reveal blind spots inside your workflow or knowing and enable you to Create more robust coding behaviors transferring ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you realized. With time, you’ll start to see styles—recurring challenges or prevalent faults—you can proactively keep away from.
In crew environments, sharing Everything you've discovered from a bug with all your friends might be Specifically potent. Whether it’s via a Slack concept, a short generate-up, or A fast information-sharing session, helping Many others stay away from the identical concern boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as essential portions of your advancement journey. After all, several of the very best builders aren't those who create great code, but those that repeatedly understand from their mistakes.
In the long run, each bug you correct provides a fresh layer on your skill set. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.